- Model Context Protocol (MCP) Server

Model Context Protocol (MCP) Server
A modular server that implements the Model Context Protocol standard, providing tools for GitHub, GitLab, Google Maps, Memory storage, and Puppeteer web automation.
Architecture
The MCP server is built with a modular architecture, where each tool is implemented as a separate module. The server provides a unified gateway that routes requests to the appropriate tool.
Features
- MCP Gateway: A unified endpoint for all tool requests following the MCP standard
- MCP Manifest: An endpoint that describes all available tools and their capabilities
- Direct Tool Access: Each tool can be accessed directly via its own API endpoints
- Modular Design: Easy to add or remove tools as needed
Included Tools
- GitHub Tool: Interact with GitHub repositories, issues, and search
- GitLab Tool: Interact with GitLab projects, issues, and pipelines
- Google Maps Tool: Geocoding, directions, and places search
- Memory Tool: Store and retrieve data persistently
- Puppeteer Tool: Take screenshots, generate PDFs, and extract content from websites
Getting Started
Prerequisites
- Python 3.8 or higher
- Node.js 14 or higher
- A Red Hat-based Linux distribution (RHEL, CentOS, Fedora) or any Linux/macOS system
Installation
-
Clone this repository:
git clone https://github.com/yourusername/mcp-server.git cd mcp-server
-
Install Python dependencies:
pip install -r requirements.txt
-
Install Node.js dependencies:
npm install
-
Create a
.env
file with your configuration:SECRET_KEY=your-secret-key DEBUG=False # GitHub configuration GITHUB_TOKEN=your-github-token # GitLab configuration GITLAB_TOKEN=your-gitlab-token # Google Maps configuration GMAPS_API_KEY=your-google-maps-api-key # Memory configuration MEMORY_DB_URI=sqlite:///memory.db # Puppeteer configuration PUPPETEER_HEADLESS=true CHROME_PATH=/usr/bin/chromium-browser
-
Start the server:
python app.py
Containerized Deployment
You can run the server using either Docker or Podman (Red Hat's container engine).
Docker Deployment
If you already have Docker and docker-compose installed:
-
Build the Docker image:
docker build -t mcp-server .
-
Run the container:
docker run -p 5000:5000 --env-file .env mcp-server
-
Alternatively, use docker-compose:
Create a
docker-compose.yml
file:version: '3' services: mcp-server: build: . ports: - "5000:5000" volumes: - ./data:/app/data env_file: - .env restart: unless-stopped
Then run:
docker-compose up -d
Podman Deployment
For Red Hat based systems (RHEL, CentOS, Fedora) using Podman:
-
Build the container image:
podman build -t mcp-server .
-
Run the container:
podman run -p 5000:5000 --env-file .env mcp-server
-
If you need persistent storage:
mkdir -p ./data podman run -p 5000:5000 --env-file .env -v ./data:/app/data:Z mcp-server
Note: The
:Z
suffix is important for SELinux-enabled systems. -
Using Podman Compose (if installed):
# Install podman-compose if needed pip install podman-compose # Use the same docker-compose.yml file as above podman-compose up -d
Using the MCP Server
MCP Gateway
The MCP Gateway is the main endpoint for accessing all tools using the MCP standard.
Endpoint: POST /mcp/gateway
Request format:
{
"tool": "github",
"action": "listRepos",
"parameters": {
"username": "octocat"
}
}
Response format:
{
"tool": "github",
"action": "listRepos",
"status": "success",
"result": [
{
"id": 1296269,
"name": "Hello-World",
"full_name": "octocat/Hello-World",
"owner": {
"login": "octocat",
"id": 1
},
...
}
]
}
MCP Manifest
The MCP Manifest describes all available tools and their capabilities.
Endpoint: GET /mcp/manifest
Response format:
{
"manifestVersion": "1.0",
"tools": {
"github": {
"actions": {
"listRepos": {
"description": "List repositories for a user or organization",
"parameters": {
"username": {
"type": "string",
"description": "GitHub username or organization name"
}
},
"returns": {
"type": "array",
"description": "List of repository objects"
}
},
...
}
},
...
}
}
Direct Tool Access
Each tool can also be accessed directly via its own API endpoints:
- GitHub:
/tool/github/...
- GitLab:
/tool/gitlab/...
- Google Maps:
/tool/gmaps/...
- Memory:
/tool/memory/...
- Puppeteer:
/tool/puppeteer/...
See the API documentation for each tool for details on the available endpoints.
Tool Documentation
GitHub Tool
The GitHub tool provides access to the GitHub API for repositories, issues, and search.
Actions:
listRepos
: List repositories for a user or organizationgetRepo
: Get details for a specific repositorysearchRepos
: Search for repositoriesgetIssues
: Get issues for a repositorycreateIssue
: Create a new issue in a repository
GitLab Tool
The GitLab tool provides access to the GitLab API for projects, issues, and pipelines.
Actions:
listProjects
: List all projects accessible by the authenticated usergetProject
: Get details for a specific projectsearchProjects
: Search for projects on GitLabgetIssues
: Get issues for a projectcreateIssue
: Create a new issue in a projectgetPipelines
: Get pipelines for a project
Google Maps Tool
The Google Maps tool provides access to the Google Maps API for geocoding, directions, and places search.
Actions:
geocode
: Convert an address to geographic coordinatesreverseGeocode
: Convert geographic coordinates to an addressgetDirections
: Get directions between two locationssearchPlaces
: Search for places using the Google Places APIgetPlaceDetails
: Get details for a specific place
Memory Tool
The Memory tool provides a persistent key-value store for storing and retrieving data.
Actions:
get
: Get a memory item by keyset
: Create or update a memory itemdelete
: Delete a memory item by keylist
: List all memory items, with optional filteringsearch
: Search memory items by value
Puppeteer Tool
The Puppeteer tool provides web automation capabilities for taking screenshots, generating PDFs, and extracting content from websites.
Actions:
screenshot
: Take a screenshot of a webpagepdf
: Generate a PDF of a webpageextract
: Extract content from a webpage
Contributing
Contributions are welcome! Here's how you can extend the MCP server:
Adding a New Tool
- Create a new file in the
tools
directory, e.g.,tools/newtool_tool.py
- Implement the tool with actions following the same pattern as existing tools
- Add the tool to the manifest in
app.py
- Register the tool's blueprint in
tools/__init__.py
License
This project is licensed under the MIT License - see the LICENSE file for details.
Acknowledgements
- Model Context Protocol for the standard specification
- Flask for the web framework
- Puppeteer for web automation



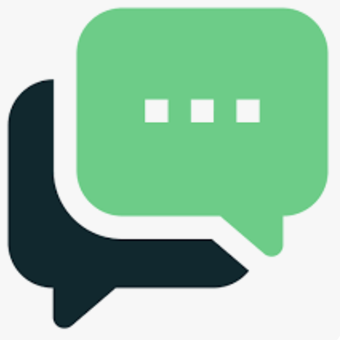
