- Browser-use MCP Client
Information
Browser-use MCP Client
A modern React application that provides a user-friendly interface for interacting with Model Context Protocol (MCP) servers through Server-Sent Events (SSE).
🎥 Demo
https://github.com/user-attachments/assets/52ab11ad-741f-4506-99ad-9f1972a3aad1
🚀 Features
- Real-time Communication: Direct SSE connection to MCP servers
- Interactive UI: Clean and responsive interface built with React and Tailwind CSS
- Theme Support: Light and dark mode with system preference detection
- Screenshot Preview: Live browser screenshots from MCP server responses
- Message History: Persistent chat history with clear message threading
- Request Management: Cancel in-progress requests and clear chat history
- Connection Management: Easy server connection configuration
📋 Prerequisites
- Node.js (v18 or later)
- pnpm (recommended package manager)
- A running MCP server for connection
- Python 3.8+ (for running the example server)
🚀 Getting Started
-
Clone the Repository
git clone <repository-url> cd browser-use-mcp-client
-
Install Dependencies
pnpm install
-
Start the Development Server
pnpm dev
-
Start the Proxy Server
./proxy/index.js
The application will be available at http://localhost:5173
💻 Usage
🤖 Example MCP Server
Here's an example of a Python-based MCP server that uses browser automation:
#!/usr/bin/env python3
import asyncio
from dotenv import load_dotenv
from typing import Awaitable, Callable
from mcp.server.fastmcp import FastMCP, Context
from browser_use import Agent, Browser, BrowserConfig
from langchain_google_genai import ChatGoogleGenerativeAI
# Load environment variables from .env file
load_dotenv()
# Initialize FastMCP server
mcp = FastMCP("browser-use")
browser = Browser(
config=BrowserConfig(
chrome_instance_path="/Applications/Google Chrome.app/Contents/MacOS/Google Chrome --remote-debugging-port=9222",
headless=True
)
)
llm = ChatGoogleGenerativeAI(model="gemini-2.0-flash")
agent = None
@mcp.tool()
async def perform_search(task: str, context: Context):
"""Perform the actual search in the background."""
async def step_handler(state, *args):
if len(args) != 2:
return
await context.session.send_log_message(
level="info",
data={"screenshot": state.screenshot, "result": args[0]}
)
asyncio.create_task(
run_browser_agent(task=task, on_step=step_handler)
)
return "Processing Request"
@mcp.tool()
async def stop_search(*, context: Context):
"""Stop a running browser agent search by task ID."""
if agent is not None:
await agent.stop()
return "Running Agent stopped"
async def run_browser_agent(task: str, on_step: Callable[[], Awaitable[None]]):
"""Run the browser-use agent with the specified task."""
global agent
try:
agent = Agent(
task=task,
browser=browser,
llm=llm,
register_new_step_callback=on_step,
register_done_callback=on_step,
)
await agent.run()
except asyncio.CancelledError:
return "Task was cancelled"
except Exception as e:
return f"Error during execution: {str(e)}"
finally:
await browser.close()
if __name__ == "__main__":
mcp.run(transport="sse")
🤝 Contributing
- Fork the repository
- Create a feature branch
- Commit your changes
- Push to the branch
- Open a Pull Request
📄 License
This project is licensed under the MIT License - see the LICENSE file for details.
Recommended Servers

MongoDB LensFull featured MCP Server for MongoDB database analysis.
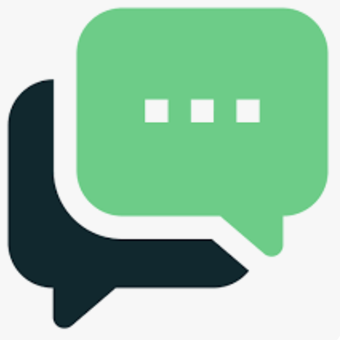
ChatSumQuery and Summarize your chat messages.

Microsoft SQL Server MCP Server (MSSQL)MS SQL MCP Server
An easy-to-use bridge that lets AI assistants like Claude directly query and explore Microsoft SQL Server databases. No coding experience required!
What Does This Tool Do?
This tool allows AI assistants to:
Discover tables in your SQL Server database
View table structures (columns, data types, etc.)
Execute read-only SQL queries safely
Generate SQL queries from natural language requests
Perplexity Web Search MCP ServerA perplexity MCP server
mcp-deepresearchlocal mcp server perplexity
Template for MCP Server

Mcp Server RagdocsAn MCP server that provides tools for retrieving and processing documentation through vector search, both locally or hosted. Enabling AI assistants to augment their responses with relevant documentation context.

GithubMCP Server for the GitHub API, enabling file operations, repository management, search functionality, and more.
Most Popular Model Context Protocol (MCP) ServersA curated list of the most popular Model Context Protocol (MCP) servers based on usage data from Smithery.ai
Nuanced MCP ServerA Model Context Protocol (MCP) server that provides call graph analysis capabilities to LLMs through the nuanced library